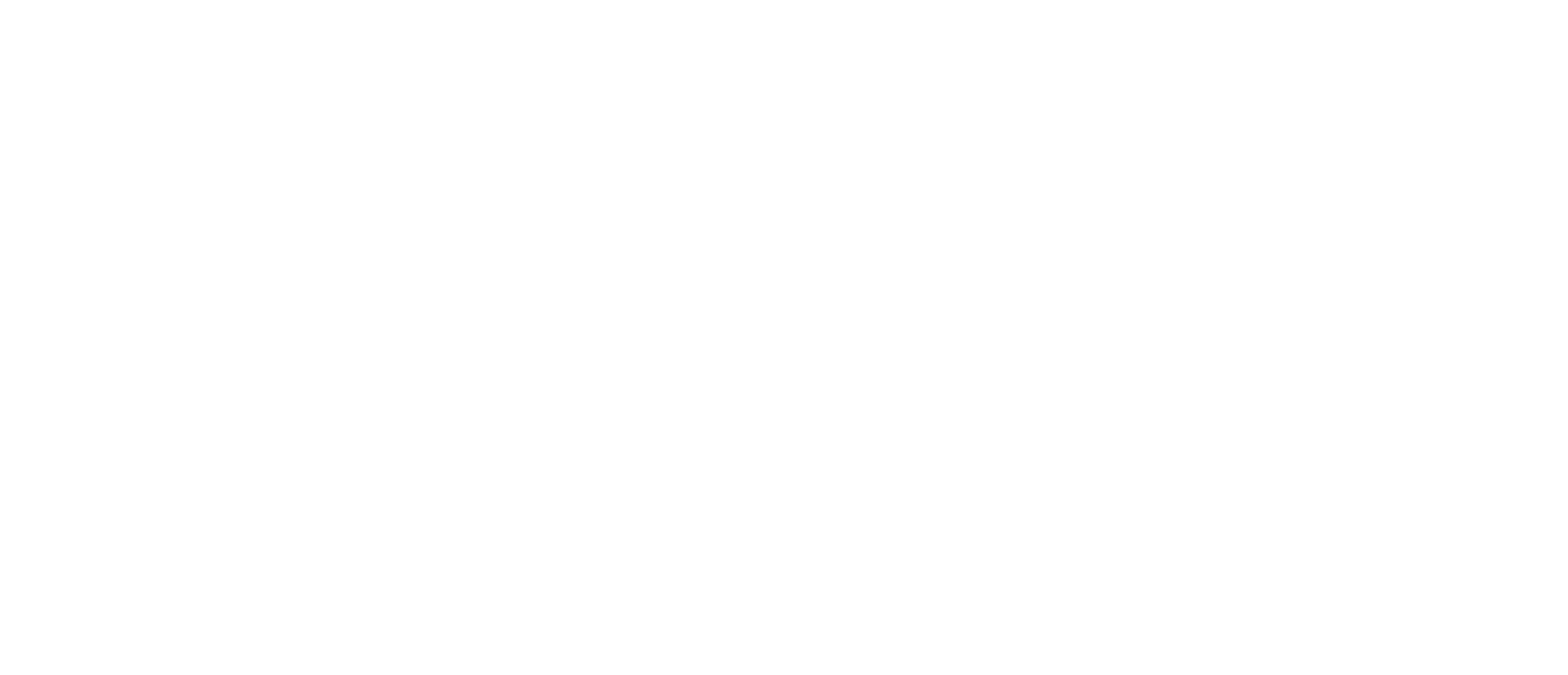
Javascript Guidelines
Object Rules
Place the opening bracket on the same line as the object name.
Use colon plus one space between each property and its value.
Use quotes around string values, not around numeric values.
Do not add a comma after the last property-value pair.
Place the closing bracket on a new line, without leading spaces.
Always end an object definition with a semicolon.
Rules
Statement Rules
Always end a simple statement with a semicolon.
General rules for complex (compound) statements:
Put the opening bracket at the end of the first line.
Use one space before the opening bracket.
Put the closing bracket on a new line, without leading spaces. Do not end a complex statement with a semicolon.
Declarations on Top: it is a good coding practice to put all declarations at the top of each script or function.
This will:
Give cleaner code
Provide a single place to look for local variables
Make it easier to avoid unwanted (implied) global variables
Reduce the possibility of unwanted re-declarations
Initialize Variables: It is a good coding practice to initialize variables when you declare them.
This will:
Give cleaner code
Provide a single place to initialize variables.
Avoid undefined values
Never Declare Number, String, or Boolean Objects
Always treat numbers, strings, or booleans as primitive values. Not as objects.
Declaring these types as objects, slows down execution speed, and produces nasty side effects.
How to use it?
Variable Names: use camelCase for identifier names (variables and functions). All names start with a letter.
Spaces Around Operators: always put spaces around operators ( = + - * / ), and after commas.
Always use semicolons: relying on implicit insertion can cause subtle, hard to debug problems. Don't do it. You're better than that.
Code Indentation: always use 4 spaces for indentation of code blocks.
Line Length < 80: for readability, avoid lines longer than 80 characters.
If a JavaScript statement does not fit on one line, the best place to break it, is after an operator or a comma.
Avoid Global Variables: Minimize the use of global variables. This includes all data types, objects, and functions. Global variables and functions can be overwritten by other scripts.
Always Declare Local Variables: All variables used in a function should be declared as local variables.
Local variables must be declared with the var keyword, otherwise they will become global variables.
Don't Use new Object()
Use () instead of new Object()
Use "" instead of new String()
Use 0 instead of new Number()
Use false instead of new Boolean()
Use [] instead of new Array()
Use /()/ instead of new RegExp()
Use function (){} instead of new Function()
Example:
var
x1 =
()
; // new object
var
x2 = " " ; // new primitive string
var
x3 = 0 ; // new primitive number
var
x4 =
false
; // new primitive boolean
var
x5 =
[ ]
; // new array object
var
x6 =
/ () /
; // new regexp object
var
x7 =
function () { }
; // new function object
Use === Comparison
The == comparison operator always converts (to matching types) before comparison. The === operator forces comparison of values and type:
Example:
var
x1 =
{ }
; // new object
0 == "" ; // true
1 == "1" ; // true
1 ==
true
; // true
0 === ""; // false
1 === "1" ; // false
1 ===
true
; // false
switch (new Date().getDay()) {
case 0:
day = "Sunday";
break;
case 1:
day = "Monday";
break;
case 2:
day = "Tuesday";
break;
case 3:
day = "Wednesday";
break;
case 4:
day = "Thursday";
break;
case 5:
day = "Friday";
break;
case 6:
day = "Saturday";
break;
default:
day = "Unknown";
}
Use Parameter Defaults
If a function is called with a missing argument, the value of the missing argument is set to undefined.
Undefined values can break your code. It is a good habit to assign default values to arguments.
End Your Switches with Defaults
Always end your switch statements with a default. Even if you think there is no need for it.
Example:
// Four-space, wrap at 80. Works with very long function names, survives
// renaming without reindenting, low on space.
goog.foo.bar.doThingThatIsVeryDifficultToExplain =
function
(veryDescriptiveArgumentNumberOne, veryDescriptiveArgumentTwo, tableModelEventHandlerProxy, artichokeDescriptorAdapterIterator) {
// ...
};
Function Arguments
When possible, all function arguments should be listed on the same line. If doing so would exceed the 80column limit, the arguments must be line-wrapped in a readable way. To save space, you may wrap as close to 80 as possible, or put each argument on its own line to enhance readability.
The indentation may be either four spaces, or aligned to the parenthesis. Below are the most common patterns for argument wrapping:
var
msg = 'This is some HTML';
Strings
Prefer single quote (') over double quotes ("). For consistency single-quotes (') are preferred to doublequotes ("). This is helpful when creating strings that include HTML:
Any Questions?
For more information or comments please write to us at cemexgo.developerscenter@cemex.com and we will gladly assist you as soon as possible.
Support Links
For more reference please visit the following links: